SpringBoot整合MyBatis实现增删改查案例完整版(…
2020-03-04 16:03:01来源:博客园 阅读 ()

SpringBoot整合MyBatis实现增删改查案例完整版(附源代码)
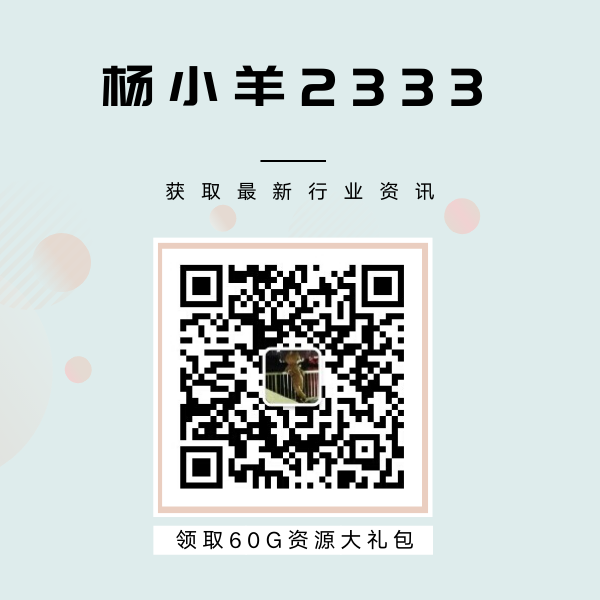
1.简介
Spring Boot是一个简化Spring开发的框架。用来监护spring应用开发,约定大于配置,去繁就简,just run 就能创建一个独立的,产品级的应用。
我们在使用Spring Boot时只需要配置相应的Spring Boot就可以用所有的Spring组件,简单的说,spring boot就是整合了很多优秀的框架,不用我们自己手动的去写一堆xml配置然后进行配置。从本质上来说,Spring Boot就是Spring,它做了那些没有它你也会去做的Spring Bean配置。
闲话少说,上图文:
2.开始
- 新建一个项目
- 选择Spring Initializr项目
- 创建项目的文件结构以及jdk的版本,本次以demo为例
- 选择项目所需要的依赖
- 修改项目名,finish完成,本次选择为修改
来看一眼pom文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
- 修改配置文件本,文不使用application.properties文件 而使用更加简洁的application.yml文件。
修改为application.yml
内容:
server:
port: 8080
spring:
datasource:
username: root
password: root
url: jdbc:mysql://localhost:3306/springboot?useUnicode=true&characterEncoding=utf-8&useSSL=true&serverTimezone=UTC
driver-class-name: com.mysql.cj.jdbc.Driver
mybatis:
mapper-locations: classpath:mapping/*Mapper.xml
type-aliases-package: com.example.entity.demo
logging:
level:
com:
example:
mapper : debug
创建表:
DROP TABLE IF EXISTS `user`;
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_general_ci NULL DEFAULT NULL,
`password` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_general_ci NULL DEFAULT NULL,
`address` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_general_ci NULL DEFAULT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 4 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_general_ci ROW_FORMAT = Dynamic;
目录结构:
UserController.java
package com.example.demo.controller;
import com.example.demo.entity.User;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.web.bind.annotation.*;
/**
* @author yn2333
* @Date 2020/03/03
*/
@RestController
@RequestMapping("/test")
public class UserController {
@Autowired
private UserService userService;
@RequestMapping(value = "/selectUserByid", produces = "application/json;charset=UTF-8", method = RequestMethod.GET)
@ResponseBody
public String GetUser(User user){
return userService.Sel(user).toString();
}
@RequestMapping(value = "/add", produces = "application/json;charset=UTF-8", method = RequestMethod.GET)
public String Add(User user){
return userService.Add(user);
}
@RequestMapping(value = "/update", produces = "application/json;charset=UTF-8", method = RequestMethod.GET)
public String Update(User user){
return userService.Update(user);
}
@RequestMapping(value = "/delete", produces = "application/json;charset=UTF-8", method = RequestMethod.GET)
public String Delete(User user){
return userService.Delete(user);
}
}
User.java
package com.example.demo.entity;
/**
* @author yn2333
* @Date 2020/03/03
*/
public class User {
private Integer id;
private String username;
private String password;
private String address;
public User(Integer id, String username, String password, String address) {
this.id = id;
this.username = username;
this.password = password;
this.address = address;
}
public Integer getId() {
return id;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
public String getAddress() {
return address;
}
public void setId(Integer id) {
this.id = id;
}
public void setUsername(String username) {
this.username = username;
}
public void setPassword(String password) {
this.password = password;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + password + '\'' +
", address='" + address + '\'' +
'}';
}
}
UserMapper.java
package com.example.demo.mapper;
import com.example.demo.entity.User;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import org.springframework.stereotype.Repository;
/**
* @author yn2333
* @Date 2020/03/03
*/
@Repository
public interface UserMapper {
User Sel(@Param("user")User user);
int Add(@Param("user")User user);
int Update(@Param("user")User user);
int Delete(@Param("user")User user);
}
UserService.java
package com.example.demo.service;
import com.example.demo.entity.User;
import com.example.demo.mapper.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* @author yn2333
* @Date 2020/03/03
*/
@Service
public class UserService {
@Autowired
UserMapper userMapper;
public User Sel(User user) {
return userMapper.Sel(user);
}
public String Add(User user) {
int a = userMapper.Add(user);
if (a == 1) {
return "添加成功";
} else {
return "添加失败";
}
}
public String Update(User user) {
int a = userMapper.Update(user);
if (a == 1) {
return "修改成功";
} else {
return "修改失败";
}
}
public String Delete(User user) {
int a = userMapper.Delete(user);
if (a == 1) {
return "删除成功";
} else {
return "删除失败";
}
}
}
DemoApplication.java
package com.example.demo;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
* @author yn2333
* @Date 2020/03/03
*/
@MapperScan("com.example.demo.mapper") //扫描的mapper
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
UserMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.demo.entity.User">
<result column="id" jdbcType="INTEGER" property="id"/>
<result column="userName" jdbcType="VARCHAR" property="username"/>
<result column="passWord" jdbcType="VARCHAR" property="password"/>
<result column="realName" jdbcType="VARCHAR" property="address"/>
</resultMap>
<select id="Sel" resultType="com.example.demo.entity.User">
select * from user where 1=1
<if test="user.id != null">
AND id = #{user.id}
</if>
</select>
<insert id="Add" parameterType="com.example.demo.entity.User">
INSERT INTO user
<trim prefix="(" suffix=")" suffixOverrides=",">
<if test="user.username != null">
username,
</if>
<if test="user.password != null">
password,
</if>
<if test="user.address != null">
address,
</if>
</trim>
<trim prefix="VALUES (" suffix=")" suffixOverrides=",">
<if test="user.username != null">
#{user.username,jdbcType=VARCHAR},
</if>
<if test="user.password != null">
#{user.password,jdbcType=VARCHAR},
</if>
<if test="user.address != null">
#{user.address,jdbcType=VARCHAR},
</if>
</trim>
</insert>
<update id="Update" parameterType="com.example.demo.entity.User">
UPDATE user
<set>
<if test="user.username != null">
username = #{user.username},
</if>
<if test="user.password != null">
password = #{user.password},
</if>
<if test="user.address != null">
address = #{user.address},
</if>
</set>
WHERE
id=#{user.id}
</update>
<delete id="Delete" parameterType="com.example.demo.entity.User">
DELETE FROM user WHERE id = #{user.id}
</delete>
</mapper>
3.测试
- 启动
- 添加:
127.0.0.1:8080/test/add?username=无极&password=123456&address=上海市
- 查询:
127.0.0.1:8080/test/selectUserByid?id=1
- 修改
127.0.0.1:8080/test/update?id=1&username=码云&password=654321&address=北京
- 删除
127.0.0.1:8080/test/delete?id=1
增、删、改、查基本测试已经通过,Navicat刷新 之后就能看到效果。
源代码以及sql文件已打包
下载方式一:https://download.csdn.net/download/weixin_44395707/12223942
下载方式二:
扫下方二维码关注公众号回复:code001即可获取
原文链接:https://www.cnblogs.com/yn869251541/p/12410532.html
如有疑问请与原作者联系
标签:
版权申明:本站文章部分自网络,如有侵权,请联系:west999com@outlook.com
特别注意:本站所有转载文章言论不代表本站观点,本站所提供的摄影照片,插画,设计作品,如需使用,请与原作者联系,版权归原作者所有
下一篇:java数据输入习题
- springboot2配置JavaMelody与springMVC配置JavaMelody 2020-06-11
- MyBatis中的$和#,用不好,准备走人! 2020-06-11
- SpringBoot 2.3 整合最新版 ShardingJdbc + Druid + MyBatis 2020-06-11
- 掌握SpringBoot-2.3的容器探针:实战篇 2020-06-11
- nacos~配置中心功能~springboot的支持 2020-06-10
IDC资讯: 主机资讯 注册资讯 托管资讯 vps资讯 网站建设
网站运营: 建站经验 策划盈利 搜索优化 网站推广 免费资源
网络编程: Asp.Net编程 Asp编程 Php编程 Xml编程 Access Mssql Mysql 其它
服务器技术: Web服务器 Ftp服务器 Mail服务器 Dns服务器 安全防护
软件技巧: 其它软件 Word Excel Powerpoint Ghost Vista QQ空间 QQ FlashGet 迅雷
网页制作: FrontPages Dreamweaver Javascript css photoshop fireworks Flash