JDK1.7和1.8的HashMap对比详解
2020-06-02 16:08:16来源:博客园 阅读 ()

JDK1.7和1.8的HashMap对比详解
HashMap是我们在编程中最常用的map,也是面试中经常考的问题,所以打算深入研究一下hashmap的源码,并且对比7和8中的不同。
一、hashmap的数据结构
hashmap的数据结构是哈希表,核心是基于哈希值的桶,而哈希桶的底层实现其实是数组,数组这种数据结构查找的时间复杂度是O(1),所以哈希表的查找、删除、插入的**平均时间复杂度**就是O(1),但是它也有一个致命的缺陷---哈希碰撞(collision),所谓碰撞,举例说明:两个数据映射出来的哈希值相同,就会发生哈希碰撞(冲突)。下面我们来看下java7和java8分别是怎么解决碰撞问题的。
二、Java 7 HashMap(不是线程安全的)
经典的哈希表的实现---**数组+链表**,即如果put到的位置有值了,如果key不一致,则会将新put进来的数据放到这个位置原有值的后面,形成链表。画了个图简单展示下:
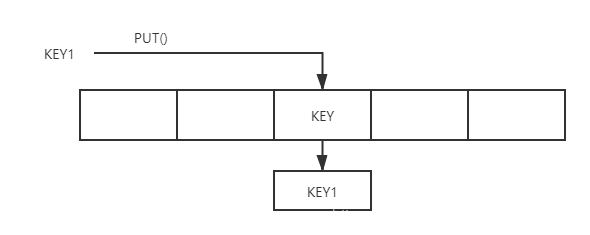
先来看几个重要的常量,简单的介绍我已经写在了下面的注释中了,其实就是把他给的英文注释简单翻译一下,他给的英文注释很清晰明了了
/**
* The default initial capacity - MUST be a power of two.
* 默认的初始化容量,必须是2的幂次方,默认容量是16(2^4)
* 疑问一:为什么必须是2的幂次方,这样赋值的目的是什么?
*/
static final int DEFAULT_INITIAL_CAPACITY = 1 << 4; // aka 16
/**
* The maximum capacity, used if a higher value is implicitly specified
* by either of the constructors with arguments.
* MUST be a power of two <= 1<<30.
* 最大容量,这个值我们一般用不上
*/
static final int MAXIMUM_CAPACITY = 1 << 30;
/**
* The load factor used when none specified in constructor.
* 加载因子,默认0.75,加载因子用来判断什么时候需要对hashmap进行resize()
*/
static final float DEFAULT_LOAD_FACTOR = 0.75f;
这里有个疑问,就是**为什么初始化容量一定是2^n**,这个我们先留着,稍后进行解答。
下面我们先去看下他的默认构造函数HashMap()
/**
* Constructs an empty <tt>HashMap</tt> with the default initial capacity
* (16) and the default load factor (0.75).
* 默认的构造函数,初始容量为16,加载因子为0.75
*/
public HashMap() {
this.loadFactor = DEFAULT_LOAD_FACTOR; // all other fields defaulted
}
当然,还有可调初始容量和加载因子的构造函数
public HashMap(int initialCapacity, float loadFactor) {
if (initialCapacity < 0)
throw new IllegalArgumentException("Illegal initial capacity: " +
initialCapacity);
if (initialCapacity > MAXIMUM_CAPACITY)
initialCapacity = MAXIMUM_CAPACITY;
if (loadFactor <= 0 || Float.isNaN(loadFactor))
throw new IllegalArgumentException("Illegal load factor: " +
loadFactor);
this.loadFactor = loadFactor;
this.threshold = tableSizeFor(initialCapacity);
}
/**
* Inflates the table
*/
private vois inflateTable(int toSize) {
iint capacity=roundUpToPowerOf2(toSize);
...//实际开辟空间存储元素
}
这里有一个需要注意的地方,当你输入的initialCapacity不是2^n,roundUpToPowerOf2(param)方法会自动将你输入的值调整为离他最大的 2的幂次方。这里说一句,并不是调用了构造函数的时候就会开辟空间,而是等到调用put方法时才会真正开辟空间存储元素(默认16)。
如何确定这个obj应该对应哪个哈希桶的索引呢?
hashcode的值有-2^(31) ~2^(31)-1,约有42亿个数,默认的哈希桶的容量是16,那么要把这些数据映射到16个桶中,我们都知道求模取余运算,但是这种方法有两个缺点:
(1)负数求模是负数,所以这里就需要将负数变成正数再取余
(2)慢!
当然,在hashmap中并不是这样的,我们看下indexFor(param)方法,这个方法就是用来解决这个问题的
```
static int indexFor(int h, int length){
return h & (length-1);
}
```
即用得到的哈希值对数组的长度进行按位与操作,下面这张图解释了为什么初始容量要是2^(n)
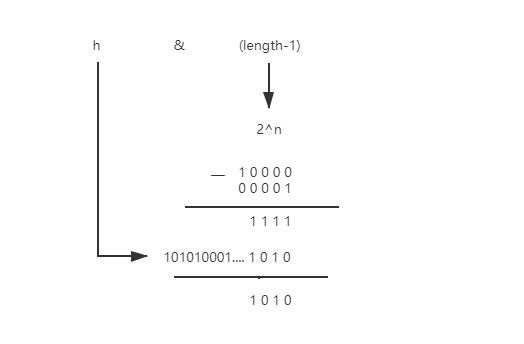
***划重点!!!***
**首先,若初始容量总为2^n,那么-1就可以得到全为1的二进制数,若(length-1)的二进制数并不总为1,h与这个二进制数进行&操作时,某一位永远为0,这个时候会出现某个桶永远不会被选中,始终为空;
其次,再进行&操作的时候,才能非常快速的拿到数组下标,并且分布还是均匀的。**
接下来,来聊一聊jdk1.7中 hash(obj) 方法:
```
final int hash(Object k){
int h=hashSeed;
if(0 != h && k instanceof String){
return sun.misc.Hashing.stringHash32((String) k);
}
h ^= k.hashCode();
h ^= (h >>> 20) ^ (h>>> 12);
return h ^ (h >>> 7) ^ (h >>> 4);
}
```
从上述贴的代码块中,可以看到先做了一次异或,而后又做了一堆异或。这个方法的注释解释为:获取这个对象的哈希值,然后进行一次补充哈希运算,得到返回结果的哈希值,他能够防御设计的非常的差的分布非常不均匀的哈希函数。如果不这样的话,就可能遇到严重的哈希碰撞。这个解释已经很清楚了,我就不再赘述了,不过这块在java8中已经被抛弃了。
然后我们来说一下**扩容**,字面意思看,就是hashmap满了,需要增大容量,直接贴代码看一下
```
/**
*新的容量是老的容量的两倍,在迁移数据的过程中涉及了一个rehash操作transfer(param)
*/
void resize(int newCapacity){
Entry[] oldTable = table;
int oldCapacity = oldTable.length;
if(oldCapacity == MAXIMUM_CAPACITY){
threshold = Integer.MAX_VALUE;
return;
}
Entry[] newTable = new Ebtry[newCapacity];
transfer(newTable, initHashSeedAsNeeded(newCapacity));
table = newTable;
threshold = (int) Math.min(newCapacity * loadFactor, MAXIMUM_CAPACITY+1);
}
```
java7中HashMap存在的问题
(1)容易碰到死锁,形成链表闭环(多线程情况下)
这里简单解释一下为什么会碰到死锁?
我先把扩容的transfer()方法代码块贴上来
```
void transfer(Entry[] newTable, boolean rehash) {
int newCapacity = newTable.length;
for (Entry<K,V> e : table) {
while(null != e) {
Entry<K,V> next = e.next;
if (rehash) {
e.hash = null == e.key ? 0 : hash(e.key);
}
int i = indexFor(e.hash, newCapacity);
e.next = newTable[i];
newTable[i] = e;
e = next;
}
}
}
```
假设当前有两个线程都在进行put操作,分别是线程A和线程B,put的哈希桶的位置已经是一个链表了,链表长度为2,第一个节点的key为key1,其next节点为key2。当线程A走到 Entry<K,V> next = e.next;这行代码时,e指向key1,next指向key2。此刻被线程B抢占了cpu,线程B在rehash后,由于使用了头插法原来的key1->key2变成了key2->key1。这个时候线程A获得了cpu的使用权,继续从之前保留的中断节点开始执行,进入while循环, e = next;就会造成死循环。
(2)潜在的安全隐患---可以通过进行构造的恶意请求引发DOS,即哈希表完全退化成链表,严重影响性能
三、Java 8 HashMap(不是线程安全的)
Java 8是对Java 7的改进,为了解决上述存在的问题
Java 8采用的是---**数组+链表/红黑树**,当链表长度增加到某阈值(8)时,链表将转变为红黑树,并且在元素减少到某阈值时,红黑树复准变为链表。
为什么变成红黑树的阈值是8呢,其实在Java 8 HashMap的注解中有提到,我把这段注释也贴了上来:
```
* Because TreeNodes are about twice the size of regular nodes, we
* use them only when bins contain enough nodes to warrant use
* (see TREEIFY_THRESHOLD). And when they become too small (due to
* removal or resizing) they are converted back to plain bins. In
* usages with well-distributed user hashCodes, tree bins are
* rarely used. Ideally, under random hashCodes, the frequency of
* nodes in bins follows a Poisson distribution
* (http://en.wikipedia.org/wiki/Poisson_distribution) with a
* parameter of about 0.5 on average for the default resizing
* threshold of 0.75, although with a large variance because of
* resizing granularity. Ignoring variance, the expected
* occurrences of list size k are (exp(-0.5) * pow(0.5, k) /
* factorial(k)). The first values are:
*
* 0: 0.60653066
* 1: 0.30326533
* 2: 0.07581633
* 3: 0.01263606
* 4: 0.00157952
* 5: 0.00015795
* 6: 0.00001316
* 7: 0.00000094
* 8: 0.00000006
* more: less than 1 in ten million
```
大致翻译下:若哈希值分布非常好的情况下,树是很少被用到的。理想情况下,在桶中节点的个数服从参数为0.5的泊松分布。即 桶中有0个值的概率是0.6...,所以这就是为什么选择阈值为8,超过8这个概率就非常小了,小于十万分之一。
下面来看代码:
几个重要的常量,中文解释直接写在上面了
```
/**
* The bin count threshold for using a tree rather than list for a
* bin. Bins are converted to trees when adding an element to a
* bin with at least this many nodes. The value must be greater
* than 2 and should be at least 8 to mesh with assumptions in
* tree removal about conversion back to plain bins upon
* shrinkage.
* 由链表转变为红黑树的阈值-8
*/
static final int TREEIFY_THRESHOLD = 8;
/**
* The bin count threshold for untreeifying a (split) bin during a
* resize operation. Should be less than TREEIFY_THRESHOLD, and at
* most 6 to mesh with shrinkage detection under removal.
* 由红黑树转变为链表的阈值-6
*/
static final int UNTREEIFY_THRESHOLD = 6;
```
还记得Java 7中的hash()算法吗,就是那个里面一堆异或的算法,在Java 8中,hash()算法只有下面几行,非常清楚简单。不过这里是将h的高16位与低16位进行了异或,在注释中提到,这样做也是为了防止低位相同高位不同的情况。
```
static final int hash(Object key) {
int h;
return (key == null) ? 0 : (h = key.hashCode()) ^ (h >>> 16);
}
```
接下来我们看下put()方法
```
final V putVal(int hash, K key, V value, boolean onlyIfAbsent,
boolean evict) {
Node<K,V>[] tab; Node<K,V> p; int n, i;
//初始化
if ((tab = table) == null || (n = tab.length) == 0)
n = (tab = resize()).length;
//找到了对应位置,且为第一个元素,则生成一个新的节点
if ((p = tab[i = (n - 1) & hash]) == null)
tab[i] = newNode(hash, key, value, null);
else {
Node<K,V> e; K k;
//如果找到的对应位置有数据,则对比key是否相同,相同则覆盖掉
if (p.hash == hash &&
((k = p.key) == key || (key != null && key.equals(k))))
e = p;
//否则的话 如果该位置的第一个节点是树节点的话,就执行树的插入操作
else if (p instanceof TreeNode)
e = ((TreeNode<K,V>)p).putTreeVal(this, tab, hash, key, value);
//否则就执行链表的插入操作
else {
for (int binCount = 0; ; ++binCount) {
if ((e = p.next) == null) {
p.next = newNode(hash, key, value, null);
//如果链表中元素个数超过TREEIFY_THRESHOLD -1 就执行转变红黑树的方法
if (binCount >= TREEIFY_THRESHOLD - 1) // -1 for 1st
treeifyBin(tab, hash);
break;
}
if (e.hash == hash &&
((k = e.key) == key || (key != null && key.equals(k))))
break;
p = e;
}
}
if (e != null) { // existing mapping for key
V oldValue = e.value;
if (!onlyIfAbsent || oldValue == null)
e.value = value;
afterNodeAccess(e);
return oldValue;
}
}
++modCount;
if (++size > threshold)
resize();
afterNodeInsertion(evict);
return null;
}
```
**扩容**
```
/**
* Initializes or doubles table size. If null, allocates in
* accord with initial capacity target held in field threshold.
* Otherwise, because we are using power-of-two expansion, the
* elements from each bin must either stay at same index, or move
* with a power of two offset in the new table.
*
* @return the table
*/
final Node<K,V>[] resize() {
Node<K,V>[] oldTab = table;
int oldCap = (oldTab == null) ? 0 : oldTab.length;
int oldThr = threshold;
int newCap, newThr = 0;
if (oldCap > 0) {
if (oldCap >= MAXIMUM_CAPACITY) {
threshold = Integer.MAX_VALUE;
return oldTab;
}
else if ((newCap = oldCap << 1) < MAXIMUM_CAPACITY &&
oldCap >= DEFAULT_INITIAL_CAPACITY)
newThr = oldThr << 1; // double threshold
}
else if (oldThr > 0) // initial capacity was placed in threshold
newCap = oldThr;
else { // zero initial threshold signifies using defaults
newCap = DEFAULT_INITIAL_CAPACITY;
newThr = (int)(DEFAULT_LOAD_FACTOR * DEFAULT_INITIAL_CAPACITY);
}
if (newThr == 0) {
float ft = (float)newCap * loadFactor;
newThr = (newCap < MAXIMUM_CAPACITY && ft < (float)MAXIMUM_CAPACITY ?
(int)ft : Integer.MAX_VALUE);
}
threshold = newThr;
@SuppressWarnings({"rawtypes","unchecked"})
Node<K,V>[] newTab = (Node<K,V>[])new Node[newCap];
table = newTab;
if (oldTab != null) {
for (int j = 0; j < oldCap; ++j) {
Node<K,V> e;
if ((e = oldTab[j]) != null) {
oldTab[j] = null;
if (e.next == null)
newTab[e.hash & (newCap - 1)] = e;
else if (e instanceof TreeNode)
((TreeNode<K,V>)e).split(this, newTab, j, oldCap);
else { // preserve order
Node<K,V> loHead = null, loTail = null;
Node<K,V> hiHead = null, hiTail = null;
Node<K,V> next;
do {
next = e.next;
if ((e.hash & oldCap) == 0) {
if (loTail == null)
loHead = e;
else
loTail.next = e;
loTail = e;
}
else {
if (hiTail == null)
hiHead = e;
else
hiTail.next = e;
hiTail = e;
}
} while ((e = next) != null);
if (loTail != null) {
loTail.next = null;
newTab[j] = loHead;
}
if (hiTail != null) {
hiTail.next = null;
newTab[j + oldCap] = hiHead;
}
}
}
}
}
return newTab;
}
```
这个注释我觉得写的非常好,告诉你在rehash时,原元素要么保持不变,要么被迁移到固定的高位,也就是说,加入现在有一个链表的元素需要迁移,那么这些元素去的地方只有两个位置,一个是原地,一个是固定高位。那这是为什么?
还记得我们之前分析的如何根据hashcode定位索引吗,我画了张图,方便理解
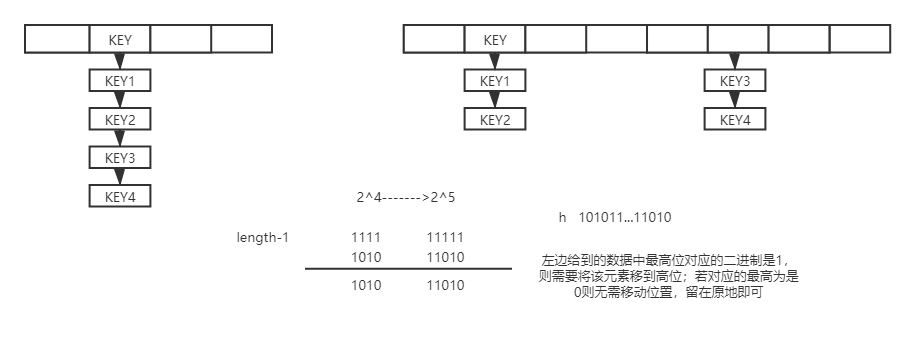
Java 8中还对迁移的元素保留的其原来位置的顺序,这样可以降低出现死锁概率,并不能避免,毕竟HashMap不是线程安全的。
那么以上。
原文链接:https://www.cnblogs.com/southtree/p/13034069.html
如有疑问请与原作者联系
标签:
版权申明:本站文章部分自网络,如有侵权,请联系:west999com@outlook.com
特别注意:本站所有转载文章言论不代表本站观点,本站所提供的摄影照片,插画,设计作品,如需使用,请与原作者联系,版权归原作者所有
- HashMap:源代码(构造方法、put、resize、get、remove、rep 2020-06-04
- HashMap1.7和1.8,红黑树原理! 2020-06-03
- HashMap理解 2020-05-24
- HashMap 的 7 种遍历方式与性能分析!(强烈推荐) 2020-05-23
- JDK1.8新特性之(三)--函数式接口 2020-05-22
IDC资讯: 主机资讯 注册资讯 托管资讯 vps资讯 网站建设
网站运营: 建站经验 策划盈利 搜索优化 网站推广 免费资源
网络编程: Asp.Net编程 Asp编程 Php编程 Xml编程 Access Mssql Mysql 其它
服务器技术: Web服务器 Ftp服务器 Mail服务器 Dns服务器 安全防护
软件技巧: 其它软件 Word Excel Powerpoint Ghost Vista QQ空间 QQ FlashGet 迅雷
网页制作: FrontPages Dreamweaver Javascript css photoshop fireworks Flash